C# Course For Automaiton
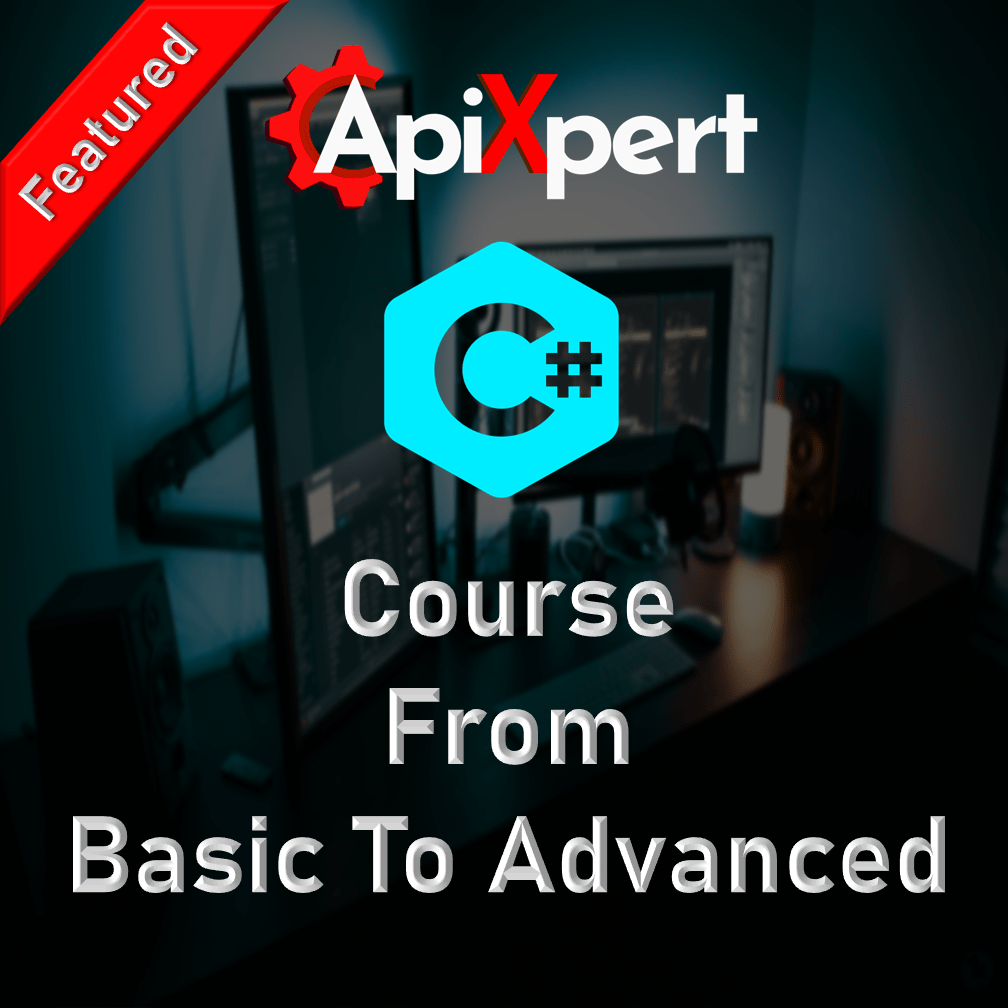
- 23 Hours
- 26 Chapters
- 60+ Topics
This C# course is specifically designed to teach you how to use C# for automating tasks in popular design and engineering software, including SolidWorks, UGNX, CATIA, and AutoCAD. Starting from the basics, you will learn fundamental programming concepts such as variables, loops, methods, and data types, and gradually advance to more complex topics like object-oriented programming (OOP), inheritance, interfaces, and exception handling. The course is tailored to automation, ensuring that you can efficiently automate repetitive tasks, create custom tools, and streamline workflows in design applications. By the end of the course, you will have the skills to develop automated solutions that can save time, reduce errors, and improve productivity in your engineering projects.
- Basic Computer Skills
- Laptop/Desktop.
- Commitment to Practice
- Participation in Community Discussions
- Individuals Looking to Adapt to New Technology and Upgrade Their Skills
- Individuals Looking for Career Change and Transition to High-Paying Job.
- Professionals in Mechanical Engineering Fields.
- Product Designers and CAD Engineers Engineering Students.
- Freelancers and Small Business Owners in Mechanical Design
- Educators in Mechanical Design and Engineering
- Corporate Teams Seeking Employee Training and Skill Development
- Engineering Students and Recent Graduates
Course Curriculum
This topic guides you through the step-by-step process of installing Visual Studio, the most powerful IDE for C# development. You'll learn how to set up the environment, select the necessary components, and configure it for seamless programming. By the end of this module, you’ll have a fully functional development setup, ready for building robust C# applications.
In this topic, you will learn how to effectively display output in C# programs, understand various data types, and declare variables to store and manipulate data. You’ll also explore arithmetic operators, which form the foundation for performing mathematical calculations. This module ensures a strong grasp of these essential concepts, preparing you for more advanced programming tasks.
In this topic, you’ll explore how to combine strings effectively using concatenation, making your outputs more dynamic and meaningful. Learn to capture user inputs to make your programs interactive. Additionally, understand angles in programming contexts and master escape sequences to handle special characters like new lines, tabs, and more. This module builds a solid foundation for writing clear and user-friendly C# programs.
This topic introduces the core concepts of decision-making in programming. Learn how to use conditional statements to control the flow of your program based on logical conditions. Understand the if-else structure for handling alternative scenarios and delve into nested if statements to manage more complex decision trees. By mastering these concepts, you'll be able to build intelligent and responsive applications.
This section covers the Switch Case Statement for handling multiple conditions efficiently in C#. You'll learn how to use switch cases as an alternative to multiple if-else statements. Additionally, it introduces Error Handling and Exception Handling, showing how to manage runtime errors using try-catch blocks and ensuring that your program can handle unexpected issues gracefully without crashing.
This section explores the different types of loops in C#, with a focus on the While Loop. You'll learn how to use loops to repeat code execution under specific conditions, enabling efficient handling of repetitive tasks. The While Loop allows for executing a block of code as long as a specified condition remains true, making it a fundamental tool in programming.
In this section, you'll learn about the For Loop and Do While Loop in C#. The For Loop is used when you know the number of iterations in advance, making it ideal for tasks with a defined range. The Do While Loop ensures that the code block is executed at least once before checking the condition, making it useful when the loop should run at least once regardless of the condition. Both loops are essential for managing repetitive tasks in your programs.
Explore Arrays, a fundamental data structure that allows you to store and manage collections of elements efficiently. Learn how to declare, initialize, and access arrays, and understand their role in organizing data in programming.
Discover the Foreach Loop, a streamlined way to iterate over arrays and collections. Understand its syntax and functionality, making it ideal for processing each element without the need for manual index management.
Dive into Multi-Dimensional Arrays, a powerful way to store data in a grid-like structure. Learn how to declare, initialize, and access elements in arrays with multiple dimensions, and understand their practical applications in handling complex data sets.
Master Nested Loops, an essential programming construct used to iterate through multi-dimensional arrays. Explore their structure, syntax, and use cases, enabling efficient processing of data in rows, columns, or other layered formats.
In this section, you'll explore String Handling Functions in C#. Learn how to manipulate and manage strings with ease using built-in functions such as Substring()
, Length()
, Replace()
, ToUpper()
, ToLower()
, Trim()
, and more. Understand how to efficiently extract, modify, and compare string data, which is essential for text processing in automation tasks with SolidWorks, UGNX, CATIA, and AutoCAD.
In this section, you will learn how to define and use Methods or Functions in C#. Methods allow you to organize your code into reusable blocks, improving efficiency and readability. You'll explore how to pass parameters, return values, and call methods from other parts of your program. Understanding how to work with methods is crucial for structuring automation scripts for SolidWorks, UGNX, CATIA, and AutoCAD, where modular and reusable code is essential for scalability and maintenance.
In this section, you'll learn about Void and Returning Methods in C#. A void method is one that does not return a value. It performs an operation or task, such as printing output or modifying data, but does not send any result back to the caller. On the other hand, a returning method sends a value back to the caller, allowing you to capture and use that result elsewhere in the program. Understanding the difference between these types of methods is key when creating automation tools for design software like SolidWorks and AutoCAD, as it helps streamline task management and data flow in your programs.
Learn how to declare static members (variables, methods, or classes) that belong to the type itself and are shared across all instances of the class. Understand how to use static members without creating an object and apply this knowledge in automation contexts like SolidWorks, AutoCAD, and UGNX.
Discover how to create and initialize objects using constructors, and learn how to use constructor overloading to define multiple constructors with different parameters for more flexible object creation.
Learn how to build class hierarchies with inheritance, explore multi-level inheritance, understand the concept of multiple inheritance, and get familiar with sealed and partial classes for advanced class management.
This topic introduces key object-oriented programming concepts in C#. You'll learn how to use virtual methods to allow derived classes to override base class behavior, abstract classes to define incomplete base classes that require derived classes to implement specific methods, and interfaces to define contracts that multiple classes can implement, ensuring flexibility and consistency in your code design.
This topic covers the usage of ref, out, and in parameters in C#, allowing you to pass variables by reference and modify or read their values efficiently. You'll also learn the difference between fields and properties in C#, exploring how fields store data directly in a class and how properties provide controlled access to that data, often with getter and setter methods.
This topic explains the differences between structs and classes in C#, highlighting how structs are value types while classes are reference types. You'll also learn about enums, which are used to define named constants for better code readability, and DateTime for handling date and time values, including formatting and manipulation techniques.
This topic covers Dynamic Link Libraries (DLLs), which allow code to be shared across multiple programs, promoting reusability and modularity. It also explores access modifiers in C#, such as public, private, protected, and internal, which define the accessibility of classes, methods, and other members in your code.
This topic introduces collections in C#, which are used to store and manage groups of related objects. It covers different types of collections, including lists, dictionaries, queues, and stacks, and explains how they help in efficiently handling data in a dynamic and flexible manner.
This topic covers the basics of file handling in C#, including reading from and writing to files. It explores how to manage files using classes like StreamReader
, StreamWriter
, and FileStream
, allowing you to manipulate data stored in text or binary files efficiently.
This section introduces you to Windows Forms Applications in C#. It covers the basics of creating graphical user interfaces (GUIs) using Windows Forms, including designing forms, adding controls (like buttons, text boxes, and labels), and handling user events. You'll learn how to build interactive desktop applications with a focus on layout and functionality.
This section introduces you to Windows Forms Applications in C#. It covers the basics of creating graphical user interfaces (GUIs) using Windows Forms, including designing forms, adding controls (like buttons, text boxes, and labels), and handling user events. You'll learn how to build interactive desktop applications with a focus on layout and functionality.
This section introduces you to Windows Forms Applications in C#. It covers the basics of creating graphical user interfaces (GUIs) using Windows Forms, including designing forms, adding controls (like buttons, text boxes, and labels), and handling user events. You'll learn how to build interactive desktop applications with a focus on layout and functionality.
- Recorded Video Lectures
- Course Handbook or eBook
- Practical Exercises and Assignments
- Discussion Forum / Community Access
- Certificate of Completion
- Resource Links and Further Reading
- Debugging and Troubleshooting Tips
The course is self-paced, so you can go through the materials at your convenience. On average, most students complete it in a few weeks depending on their learning speed and practice time.
You’ll have access to community discussions, and technical support to ensure a smooth learning experience.
There is no internship provided if you enroll in the C# course alone. However, if you take any course alongside C#—such as SolidWorks API, NX Open, CATIA Automation, or AutoCAD Automation—you will be eligible for an internship.
Absolutely! While a basic understanding of SolidWorks modeling is needed, no programming knowledge is required. The course is designed for beginners and professionals alike.
This C# course can be integrated with various design and automation software, including SolidWorks, UGNX, CATIA, AutoCAD, and more, enabling you to automate tasks and enhance workflows within these platforms.
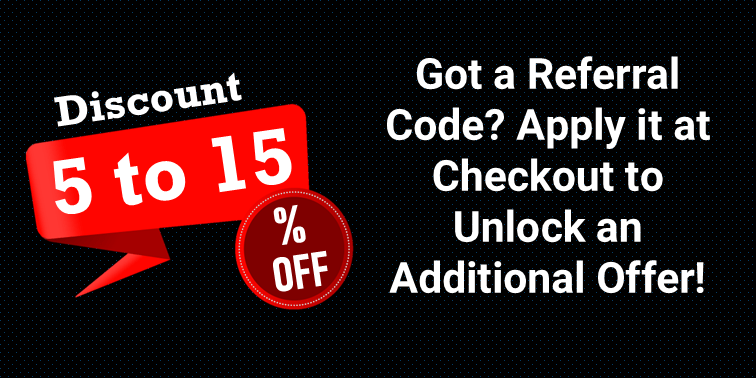